Scripts and tips for debugging code in PHP, MySQL connection and mail()
When you look for the bug, you find it.
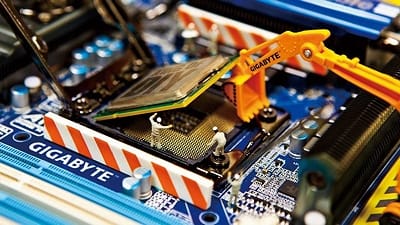
Enable PHP error reporting
You will get an extremely detailed error log with the contents of all variables. Paste the above code at the beginning of your script, just after “<?php”
Enabling PHP error reporting<?php error_reporting(E_ALL); // Enable reporting of PHP errors. You can ignore this line if you're using PHP 8.2 or later $variables = get_defined_vars(); // Gives the contents and values of all variables in the current scope $var_ignore = array("GLOBALS", "_ENV", "_SERVER"); // Determines the vars not to be displayed echo "<strong>Status of the variables on the line : " . __LINE__ . " in the file : " . __FILE__ . "</strong><br />\n"; $nom_fonction = __FUNCTION__; if ($nom_fonction !== '') { // Check if __FUNCTION__ is not empty echo "<strong>In the function : $nom_fonction</strong><br />\n"; } else { echo "<strong>Outside of a function :</strong><br />\n"; } foreach ($variables as $key => $value) { if (!in_array($key, $var_ignore) && str_contains($key, "HTTP") === false) { echo "<pre>"; echo ("$" . $key . " => "); print_r($value); echo "</pre>\n"; } }
Check the connection to its MySQL database
Create a file that you will call for example debug_mysql.php, then upload it to your server. Fill in your MySQL database connection parameters. If the script says that there is an entry and that it contains "Anne NONYME", then you have no connection problem.
You can take this PHP file here: sql-test-en.zip (1.7 KB)
Debugging MySQL database connection:<?php /* Created by "Abogil", create a debug.php file */ // Enable PHP error logging error_reporting(E_ALL); // ****** Configuration Start ****** $DBhost = "server"; // SQL server address $DBName = "name"; // SQL database name $DBowner = "username"; // SQL username $DBpw = "password"; // SQL password // ****** Configuration End ****** try { // Connect to the database $fh_db = mysqli_connect($DBhost, $DBowner, $DBpw, $DBName); if (!$fh_db) { throw new Exception( "Error connecting to the database: " . mysqli_connect_error(), ); } else { echo "1- Connection to the database = <strong>OK</strong> <br />\n"; } } catch (Exception $e) { echo "1- Error connection to the database = <strong>" . $e->getMessage() . "</strong> <br /> <br />\n"; } try { // Select the database $sel = mysqli_select_db($fh_db, $DBName); if (!$sel) { throw new Exception( "Error selecting the database: " . mysqli_error($fh_db), ); } else { echo "2- Access to the database $DBName = <strong>OK</strong> <br />\n"; } } catch (Exception $e) { echo "2- Error access to the database $DBName = <strong>" . $e->getMessage() . "</strong> <br /> <br />\n"; } // Create the table Tab_test_DB $sql_query = "CREATE TABLE `Tab_test_DB` ( `Id` int(11) NOT NULL auto_increment, `NOM` varchar(32) NOT NULL default '', `PRENOM` varchar(32) NOT NULL default '', PRIMARY KEY (`Id`)) ENGINE=MyISAM DEFAULT CHARSET=utf8"; try { // Execute the query $result_query = mysqli_query($fh_db, $sql_query); if (!$result_query) { throw new Exception( "Error creating the table: " . mysqli_error($fh_db), ); } else { echo "3- Creation of the table Tab_test_DB = <strong>OK</strong> <br />\n"; } } catch (Exception $e) { echo "3- Error creating the table $sql_query = <strong>$sql_query</strong> <br /> $result_query = <strong>" . $e->getMessage() . "</strong> <br />\n"; } // Add a record $sql_query = "INSERT INTO `Tab_test_DB` values ('','NONYME','Anne')"; try { // Execute the query $result_query = mysqli_query($fh_db, $sql_query); if (!$result_query) { throw new Exception("Error adding a record: " . mysqli_error($fh_db)); } else { echo "4- Addition of a record = <strong>OK</strong> <br />\n"; } } catch (Exception $e) { echo "4- Error adding of a record $sql_query = <strong>$sql_query</strong> <br /> $result_query = <strong>" . $e->getMessage() . "</strong> <br />\n"; } // Select from the table Tab_test_DB $sql_query = "SELECT Id, NOM, PRENOM FROM `Tab_test_DB` WHERE NOM LIKE 'NONYME'"; try { // Execute the query $result_query = mysqli_query($fh_db, $sql_query); if (!$result_query) { throw new Exception("Error selecting data: " . mysqli_error($fh_db)); } else { echo "5- The column <strong>NOM</strong> containing the value <strong>NONYME</strong> has been found. <br />\n"; } } catch (Exception $e) { echo "5- Error finding a value $sql_query = <strong>$sql_query</strong> <br /> $result_query = <strong>" . $e->getMessage() . "</strong> <br />\n"; } $sql_query = "SELECT Id, NOM, PRENOM FROM `Tab_test_DB` ORDER BY NOM"; try { // Execute the query $result_query = mysqli_query($fh_db, $sql_query); if (!$result_query) { throw new Exception("Error selecting data: " . mysqli_error($fh_db)); } $result_count_row_get = mysqli_num_rows($result_query); } catch (Exception $e) { echo "5- Error selecting a colon $sql_query = <strong>$sql_query</strong> <br /> $result_query = <strong>" . $e->getMessage() . "</strong> <br />\n"; } echo "5- Number of results found = <strong>$result_count_row_get</strong> <br />\n"; mysqli_free_result($result_query); // Drop the table Tab_test_DB $sql_query = "DROP TABLE IF EXISTS `Tab_test_DB`"; try { // Execute the query $result_query = mysqli_query($fh_db, $sql_query); if (!$result_query) { throw new Exception( "Error dropping the table: " . mysqli_error($fh_db), ); } else { echo "6- Dropping the table Tab_test_DB = <strong>OK</strong> <br />\n"; } } catch (Exception $e) { echo "6- $sql_query = <strong>$sql_query</strong> <br /> $result_query = <strong>" . $e->getMessage() . "</strong> <br />\n"; } // Close the database connection mysqli_close($fh_db); ?>
Testing the sending of messages via mail() function
Sometimes the messages don't come through. Either they are not sent because the server is down, or they are lost somewhere, or the script is badly coded. Here is a PHP script that works, it will help you test the situation. Create a file message.php and copy the code below.
You can get this PHP file here: courrier-test-en.zip (1.3 KB)
Test mail() function<?php // TEST PHP MAIL FUNCTION // CREATE A FILE email.php declare(strict_types=1); // Enable strict types // *** Configure $to = "adresse@machin.truc"; $from = "postmaster@bidule.truc"; // *** Leave as is $day = date("d-m-Y"); $time = date("H:i"); $subject = "Mail test - $day $time"; $htmlContent =<<<HTML <html> <head> <title>Subject</title> </head> <body> Simple HTML mail using PHP mail() function.<br> <b>$subject</b> <br> </body> </html> HTML; $headers = [ "MIME-Version: 1.0", "Content-Transfer-Encoding: 8bit", "Content-type: text/html; charset=utf-8", "From: $from", // "Disposition-Notification-To: $from", // receipt notification ]; try { $mail_send_verification = mail($to, $subject, $htmlContent, implode("\n", $headers)); if ($mail_send_verification === false) { throw new RuntimeException("Mail sending error"); } echo " *** Mail sending verification=$mail_send_verification - Mail sent successfully to $to from $from with subject: $subject\n"; } catch (Throwable $e) { echo " ### Mail sending verification=$mail_send_verification - Mail sending error: " . $e->getMessage() . "\n"; }
“If-” you'll be a Man…
Re-discover Rudyard Kipling's famous poem “If- you'll be a Man, my son” which has inspired so many generations. It celebrates the courage to overcome hardship.
I've decided to succeed
I have put together 10 tried and tested tips for finding the will to successfully achieve your ambitions and overcome obstacles in your personal and professional life.
Gourmet treats
Exquisite recipes for mini-cakes (madeleines, financiers, biscuits, cakes, muffins) and other delicacies (croissants, brioche, traditional cakes…).